The Java environment allows the programmer to take advantage of Java libraries for sound and to benefit from object-oriented programming features like encapsulation, inheritance, and interfaces. In this chapter, we are going to use the package javax.sound.sampled. This package provides functionality to capture, mix, and play sounds with classes such as SourceDataLine, AudioFormat, AudioSystem, and LineUnvailableException.
Program 2.5 uses a SourceDataLine object. This is the object to which we write audio data. Before doing that, we must set up the data line object with a specified audio format object. (See line 30.) The AudioFormat class specifies a certain arrangement of data in the sound stream, including the sampling rate, sample size in bits, and number of channels. A SourceDataLine object is created with the specified format, which in the example is 44,100 samples per second, eight bits per sample, and one channel for mono. With this setting, the line gets the required system resource and becomes operational. After the SourceDataLine is opened, data is written to the mixer using a buffer that contains data generated by a sine function. Notice that we don’t directly access the Sound Device because we are using a SourceDataLine object to deliver data bytes to the mixer. The mixer mixes the samples and finally delivers the samples to an audio output device on a sound card.
import javax.sound.sampled.AudioFormat; import javax.sound.sampled.AudioSystem; import javax.sound.sampled.SourceDataLine; import javax.sound.sampled.LineUnavailableException; public class ExampleTone1{ public static void main(String[] args){ try { ExampleTone1.createTone(262, 100); } catch (LineUnavailableException lue) { System.out.println(lue); } } /** parameters are frequency in Hertz and volume **/ public static void createTone(int Hertz, int volume) throws LineUnavailableException { /** Exception is thrown when line cannot be opened */ float rate = 44100; byte[] buf; AudioFormat audioF; buf = new byte[1]; audioF = new AudioFormat(rate,8,1,true,false); //sampleRate, sampleSizeInBits,channels,signed,bigEndian SourceDataLine sourceDL = AudioSystem.getSourceDataLine(audioF); sourceDL = AudioSystem.getSourceDataLine(audioF); sourceDL.open(audioF); sourceDL.start(); for(int i=0; i<rate; i++){ double angle = (i/rate)*Hertz*2.0*Math.PI; buf[0]=(byte)(Math.sin(angle)*volume); sourceDL.write(buf,0,1); } sourceDL.drain(); sourceDL.stop(); sourceDL.close(); } }
Program 2.5 A simple sound generating program in Java
This program illustrates a simple of way of generating a sound by using a sine wave and the javax.sound.sampled library. If we change the values of the createTone procedure parameters, which are 262 Hz for frequency and 100 for volume, we can produce a different tone. The second parameter, volume, is used to change the amplitude of the sound. Notice that the sine function result is multiplied by the volume parameter in line 40.
Although the purpose of this section of the book is not to demonstrate how Java graphics classes are used, it may be helpful to use some basic plot features in Java to generate sine wave drawings. An advantage of Java is that it facilitates your control of windows and containers. We inherit this functionality from the JPanel class, which is a container where we are going to paint the sine wave generated. Program 2.6 is a variation of Program 2.5. It produces a Java Window by using the procedure paintComponent. This sine wave generated again has a frequency of 262 Hz and a volume of 100.
import javax.sound.sampled.AudioFormat; import javax.sound.sampled.AudioSystem; import javax.sound.sampled.SourceDataLine; import javax.sound.sampled.LineUnavailableException; import java.awt.*; import java.awt.geom.*; import javax.swing.*; public class ExampleTone2 extends JPanel{ static double[] sines; static int vol; public static void main(String[] args){ try { ExampleTone2.createTone(262, 100); } catch (LineUnavailableException lue) { System.out.println(lue); } //Frame object for drawing JFrame frame = new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(new ExampleTone2()); frame.setSize(800,300); frame.setLocation(200,200); frame.setVisible(true); } public static void createTone(int Hertz, int volume) throws LineUnavailableException { float rate = 44100; byte[] buf; buf = new byte[1]; sines = new double[(int)rate]; vol=volume; AudioFormat audioF; audioF = new AudioFormat(rate,8,1,true,false); SourceDataLine sourceDL = AudioSystem.getSourceDataLine(audioF); sourceDL = AudioSystem.getSourceDataLine(audioF); sourceDL.open(audioF); sourceDL.start(); for(int i=0; i<rate; i++){ double angle = (i/rate)*Hertz*2.0*Math.PI; buf[0]=(byte)(Math.sin(angle)*vol); sourceDL.write(buf,0,1); sines[i]=(double)(Math.sin(angle)*vol); } sourceDL.drain(); sourceDL.stop(); sourceDL.close(); } protected void paintComponent(Graphics g) { super.paintComponent(g); Graphics2D g2 = (Graphics2D)g; g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); int pointsToDraw=4000; double max=sines[0]; for(int i=1;i<pointsToDraw;i++) if (max<sines[i]) max=sines[i]; int border=10; int w = getWidth(); int h = (2*border+(int)max); double xInc = 0.5; //Draw x and y axes g2.draw(new Line2D.Double(border, border, border, 2*(max+border))); g2.draw(new Line2D.Double(border, (h-sines[0]), w-border, (h-sines[0]))); g2.setPaint(Color.red); for(int i = 0; i < pointsToDraw; i++) { double x = border + i*xInc; double y = (h-sines[i]); g2.fill(new Ellipse2D.Double(x-2, y-2, 2, 2)); } } }
Program 2.6 Visualizing the sound waves in a Java program
If we increase the value of the frequency in line 18 to 400 Hz, we can notice how the number of cycles increases, as shown in Figure 2.57. On the other hand, by increasing the volume, we obtain a higher amplitude for each frequency.
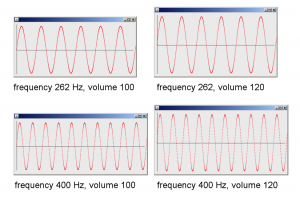
[wpfilebase tag=file id=77 tpl=supplement /]
We can also create square, triangle, and sawtooth waves in Java by modifying the for loop in lines 49 to 52. For example, to create a square wave, we may change the for loop to something like the following:
for(int i=0; i<rate; i++){ double angle1 = i/rate*Hertz*1.0*2.0*Math.PI; double angle2 = i/rate*Hertz*3.0*2.0*Math.PI; double angle3 = i/rate*Hertz*5.0*2.0*Math.PI; double angle4 = i/rate*Hertz*7.0*2.0*Math.PI; buf[0]=(byte)(Math.sin(angle1)*vol+ Math.sin(angle2)*vol/3+Math.sin(angle3)*vol/5+ Math.sin(angle4)*vol/7); sdl.write(buf,0,1); sines[i]=(double)(Math.sin(angle1)*vol+ Math.sin(angle2)*vol/3+Math.sin(angle3)*vol/5+ Math.sin(angle4)*vol/7); }
This for loop produces the sine wave shown in Figure 2.58. This graph doesn’t look like a perfect square wave, but the more harmonic frequencies we add, the closer we get to a square wave. (Note that you can create these waveforms more exactly by adapting the Octave programs above to Java.)
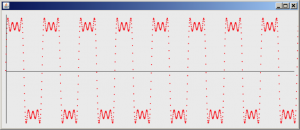